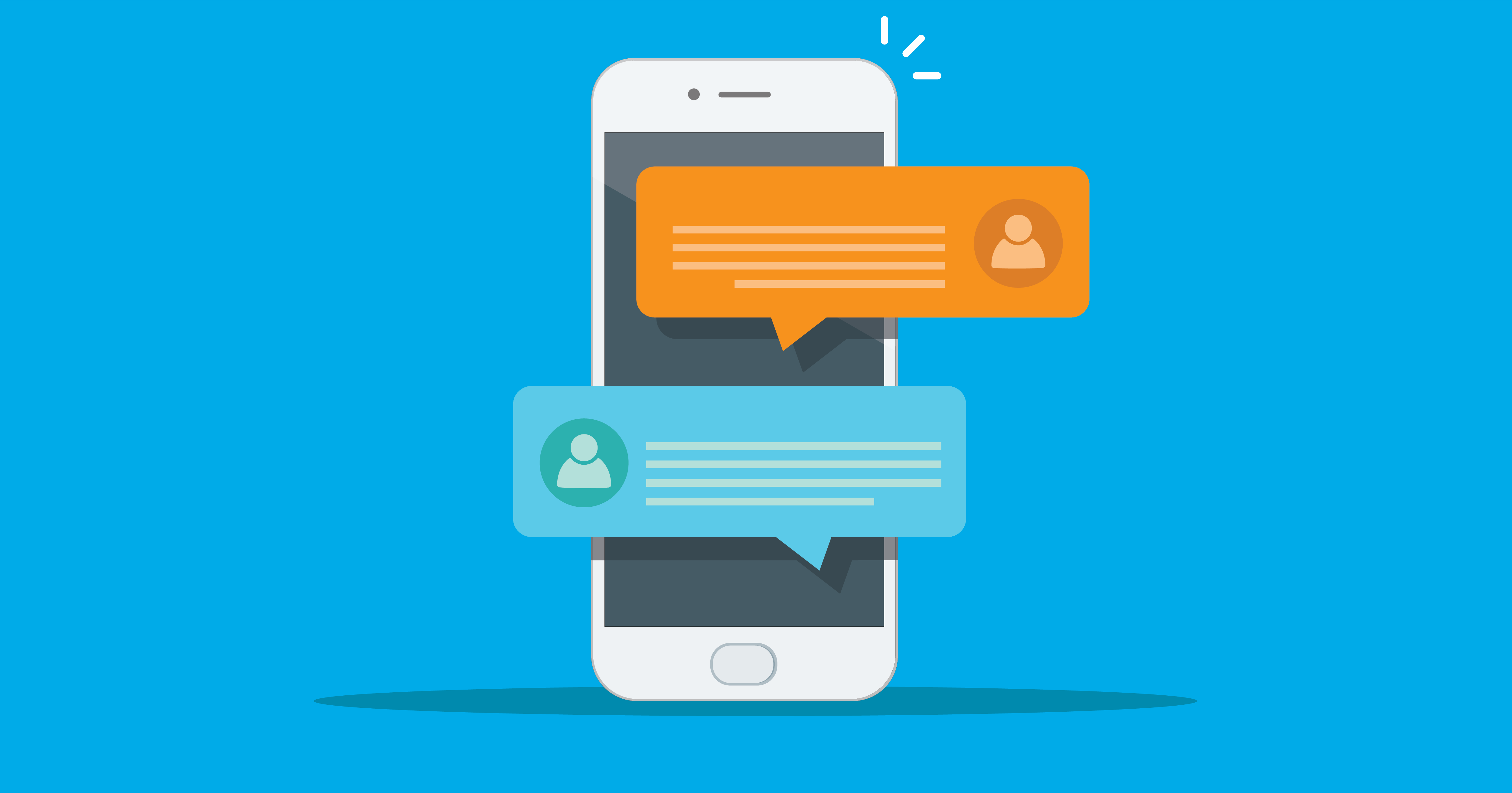
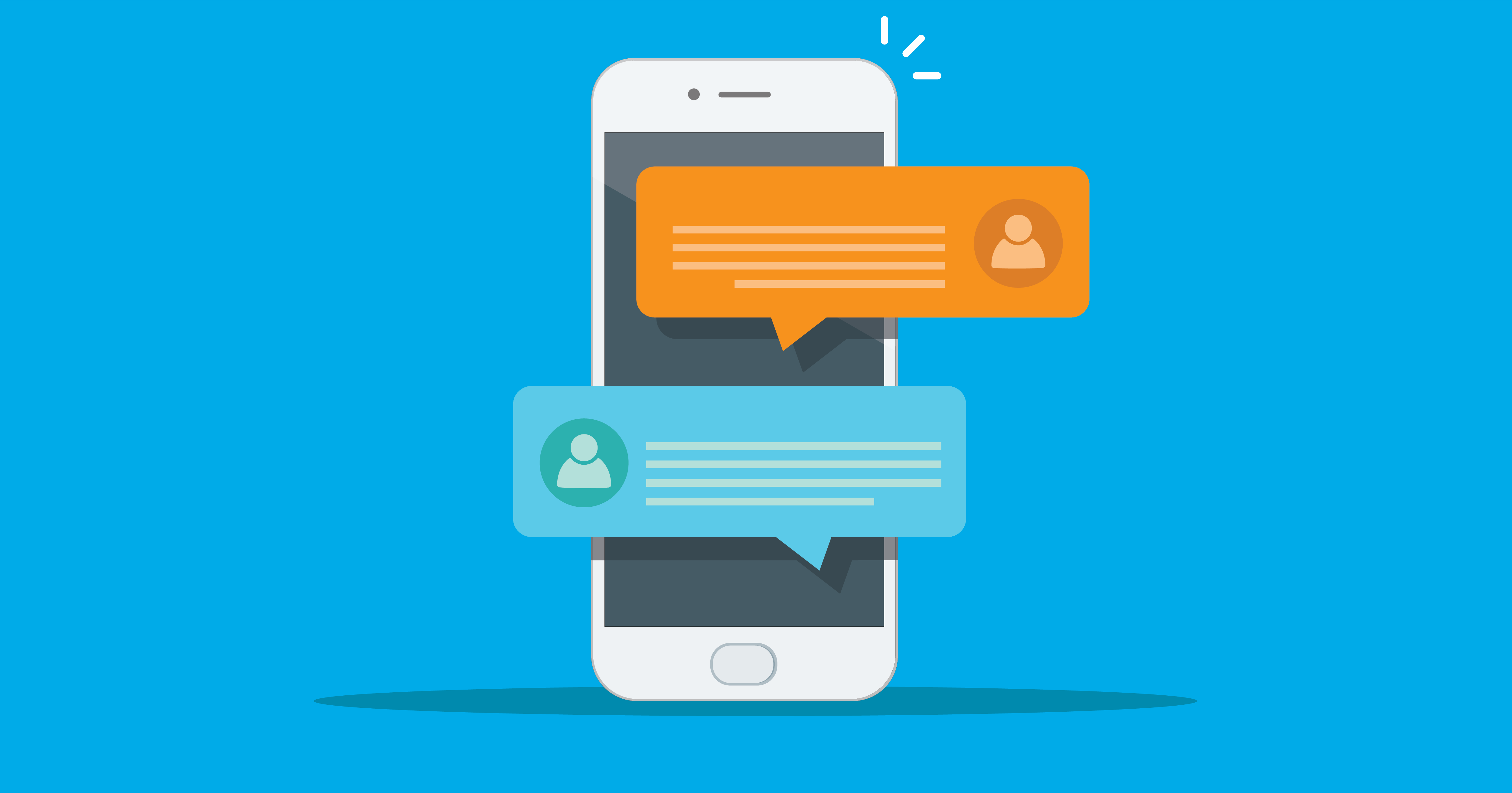
When you look at the different applications LiveSwitch powers, you will see that the focus of each application is real time audio and video. While that is a powerful tool and will remain the focus of LiveSwitch, it is not always sufficient for every use case. To help you achieve your use case, LiveSwitch offers data channels that allow you to send any type of data you may need from one user to another.
One specific application of data channels is for chat. Much like the rest of the features available in the LiveSwitch SDK, you can do a very simple implementation of chat or customize it as much as needed for your use case.
The good news is that there is no additional setup required to enable chat for a LiveSwitch session. All the messaging happens using the channel object that is created when you register your client.
There are 2 blocks of code that you will need to add to your application. This first block is the minimum code required to send a chat message.
channel.sendMessage("Here is my chat message")
In this example, the text will be sent to all other clients registered on the channel, however, there is no information on who sent the message. We can create a simple object to hold different pieces of information to make our chat message more detailed.
var message = {
from: displayName,
message: "Here is my chat message."
}
channel.sendMessage(JSON.stringify(message))
In this example, we create a message object with two properties, from and message. We can now add the sender information along with the chat message. The only added step is to take the JSON object and turn it into a string using the JSON.stringify() function.
You can continue to add data based on your use case to the chat messages this way.
Additionally you can add encryption to your messages by passing the stringified object through an encryption function before calling channel.sendMessage().
The next block of code you need to add is a handler for incoming messages. In this block, you will define what happens when a new message appears on the channel. This will also include the messages you send!
channel.addOnMessage(function(sender: ls.ClientInfo, message: string){
const data = JSON.parse(message);
console.log("From: " + data.from + " Message: " + data.message)
})
Please note that any additional encryption or processing you do before sending the messaging will need to be reversed in this handler function. Once you have the data object above, you can display the contents in whatever way you like within your application. You have access to the ClientInfo object from the sender (this does not include the display name) and the message itself. It is recommended that you display your own messages differently than how you display messages from others.
In order to see this in action, take a look at our open source Vue example application. You can see a running demo of the application and the source code. You can find the majority of the chat logic inside SDKPlugin.ts and the rest in InCall.vue.
Additionally, if you would like to try out adding chat to your application, sign up for our free 30-day trial.
Need assistance in architecting the perfect WebRTC application? Let our team help out! Get in touch with us today!