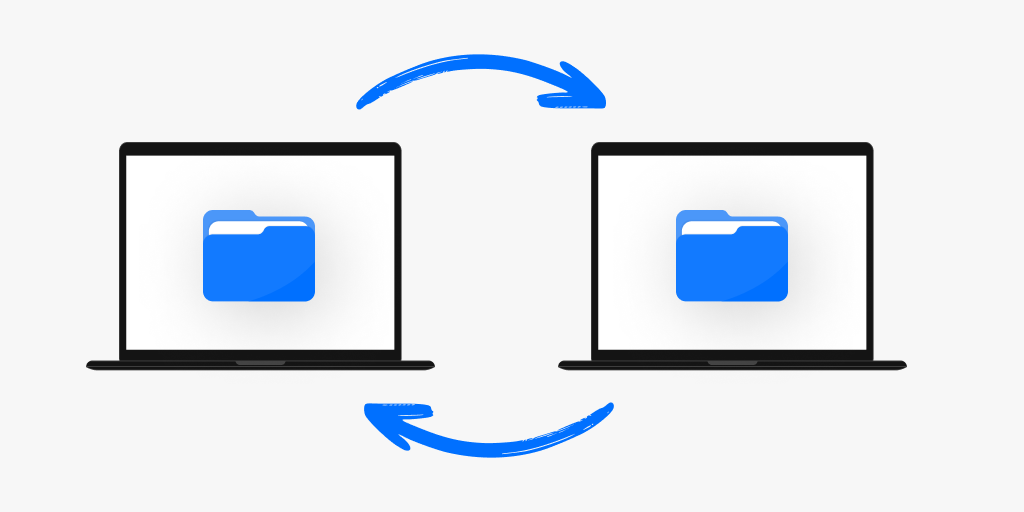
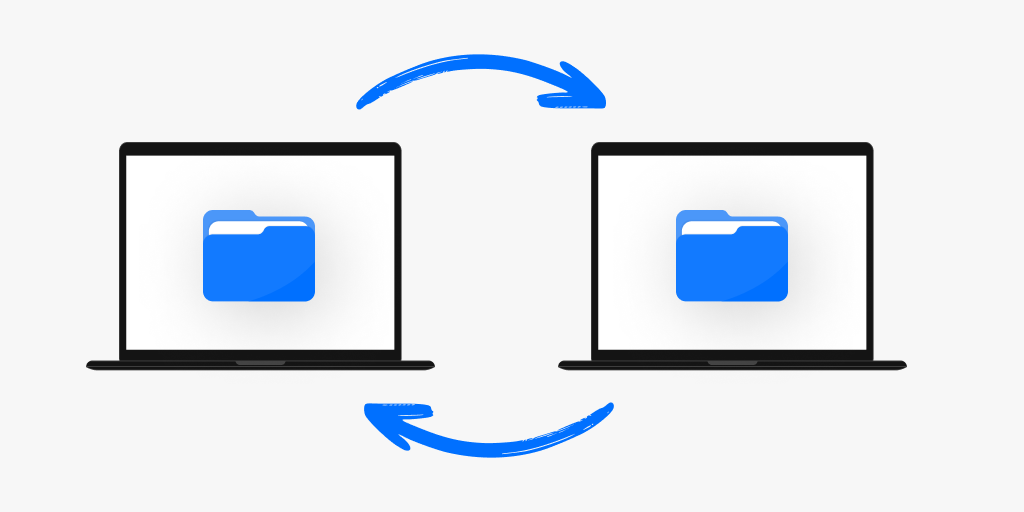
In this sample console application, we will explore how to send a file from one connection to another using DataStreams and a DataChannel in LiveSwitch. The process is straightforward and involves establishing connections, creating data channels, and implementing file transfer functionality. By following this guide, you'll be able to send files efficiently over data channels in no time.
Before running the application, make sure to complete the following steps:
<APPLICATIONID>
with your actual application ID.<SHAREDSECRET>
with your shared secret.FM.LiveSwitch
NuGet package (only the FM.LiveSwitch
package is required for this example).using FM.LiveSwitch;
var client1 = new Client("https://cloud.liveswitch.io/", "<APPLICATIONID>");
var client2 = new Client("https://cloud.liveswitch.io/", "<APPLICATIONID>");
var token1 = Token.GenerateClientRegisterToken(client1, new ChannelClaim[] { new ChannelClaim("byteDataTransferTest") }, "<SHAREDSECRET>");
var token2 = Token.GenerateClientRegisterToken(client2, new ChannelClaim[] { new ChannelClaim("byteDataTransferTest") }, "<SHAREDSECRET>");
var channels = await client1.Register(token1);
var channel1 = channels[0];
channels = await client2.Register(token2);
var channel2 = channels[0];
var dataChannel1 = new DataChannel("test");
var dataChannel2 = new DataChannel("test");
var dataStream1 = new DataStream(dataChannel1);
var dataStream2 = new DataStream(dataChannel2);
var upConnection = channel1.CreateSfuUpstreamConnection(dataStream1, "mediaId");
var downConnection = channel2.CreateSfuDownstreamConnection("mediaId", dataStream2);
bool hasWrittenFile = false;
Func<bool> selector = () =>
{
while (!hasWrittenFile)
{
Thread.Sleep(1000);
}
var txt = File.ReadAllText("Received.txt");
Console.WriteLine("File Written.");
Console.WriteLine(txt);
return true;
};
var task = new Task<bool>(selector);
dataChannel1.OnStateChange += (DataChannel dc) =>
{
if (dc.State == DataChannelState.Connected)
{
var file = File.ReadAllBytes("SendMe.txt");
var wrapper = DataBuffer.Wrap(file);
dc.SendDataBytes(wrapper);
}
};
dataChannel2.OnReceive += (DataChannelReceiveArgs args) =>
{
if (args.DataBytes != null)
{
File.WriteAllBytes("Received.txt", args.DataBytes.ToArray());
hasWrittenFile = true;
}
};
await downConnection.Open();
await upConnection.Open();
task.Start();
Task.WaitAny(new Task[] { task });
In the sample code, we establish two clients, register them with the LiveSwitch Gateway, create DataChannels for communication, and set up the necessary connections.
To send a file, we convert it into bytes and wrap them in a data buffer using the DataBuffer.Wrap
method. Then, we use the SendDataBytes
method of the DataChannel to send the data buffer containing the file. On the receiving side, we handle the OnReceive
event of the DataChannel and extract the received bytes from the DataChannelReceiveArgs
object. Finally, we write the received bytes to a file.
Note: Besides strings, DataChannels in LiveSwitch can only transmit bytes. Therefore, to send a file, it needs to be converted into bytes and wrapped in a data buffer before being sent using the SendDataBytes
method of the DataChannel.
Please note that the full project files for quick running are available here.
Feel free to customize the code according to your specific requirements and explore the possibilities of file sharing through DataChannels.
Need assistance in architecting the perfect WebRTC application? Let our team help out! Get in touch with us today!